In this blog post, you will find the HackerRank Python Basic certification Solutions. The answers to questions are:-
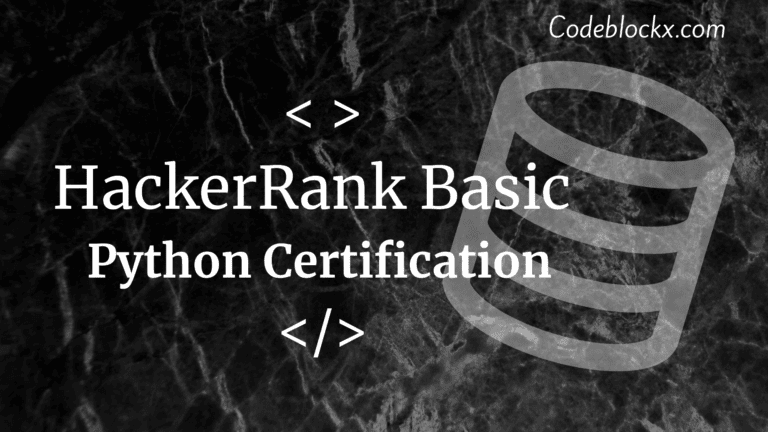
Hackerrank Python Certification Solution
Q1:- Shape Classes with Area
import math
class Rectangle:
def __init__(self, l, w):
self.length = l
self.width = w
def area(self):
return self.length * self.width
class Circle:
def __init__(self, r):
self.radius = r
def area(self):
return math.pi * self.radius ** 2
if __name__ == “__main__”:
q = int(input())
queries = []
for _ in range(q):
args = input().split()
shape_name, params = args[0], list(map(int, args[1:]))
if shape_name == “rectangle”:
a, b = params[0], params[1]
shape = Rectangle(a, b)
elif shape_name == “circle”:
r = params[0]
shape = Circle(r)
else:
raise ValueError(“invalid shape type”)
area_result = shape.area()
print(“{:.2f}”.format(area_result))
Q2:- Missing Characters
def missingCharacters(s):
# Create sets for all digits and lowercase English letters
all_digits = set(‘0123456789’)
all_letters = set(‘abcdefghijklmnopqrstuvwxyz’)
# Convert the input string to lowercase and create a set from its characters
s_set = set(s.lower())
# Find the missing digits and characters
missing_digits = sorted(all_digits – s_set)
missing_letters = sorted(all_letters – s_set)
# Combine the missing digits and letters in the desired order
result = ”.join(missing_digits + missing_letters)
return result
if __name__ == ‘__main__’:
s = ‘8hypotheticall024y6wxz’
result = missingCharacters(s)
print(result)
Hope this blog post was helpful to you and you have got the answer to your question. Thank you for visiting our blog. If you have any doubts about any coding questions then let us know in the comments section we will answer them as soon as possible. Till then check out our more blog posts.