Hello Coders, In this blog post, You will learn to write the code to find the perimeter of the circle in Python. The code for this program is very easy and you will understand it instantly. We have written this code in three different ways. Let’s discuss them in detail:-
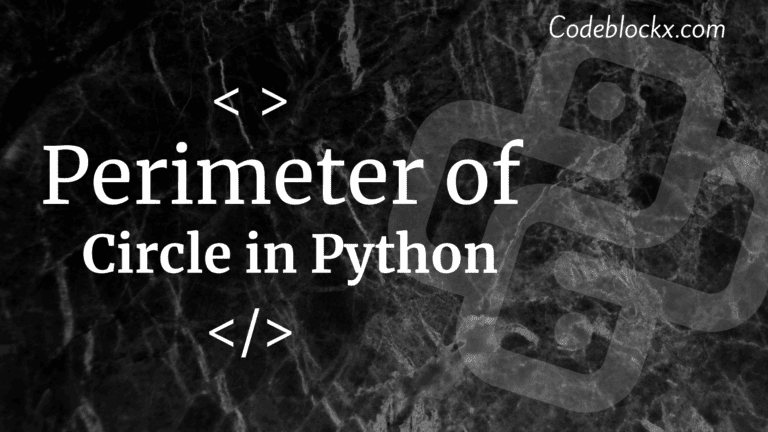
Perimter of Circle
Perimeter = 2 * Pi * R
Where Pi = 3.14 and R is radius of circle
Ways to Find Perimeter of Circle in Python:-
1. Simple Way:-
This is the simplest code for the perimeter of a circle. In this code, we have taken the radius as input from the user in float so that the radius in decimal can also be answered. Then we have defined the value of pi as 3.14. After this, we applied the formula of the perimeter, and the answer was printed on the output screen with the help of the print function.
Simple.py
OUTPUT
Enter the radius of circle: 5
The perimeter of circle is: 31.400000000000002
2. Using Math Library:-
In this method, We will import a math library that is already built in Python. The use of this library is that now there is no need to enter the value pi as it will now automatically update. After importing the library we will input the value of the radius from the user and enter the value of the perimeter of a circle using the formula 2*pi*r. After this, we will print the perimeter of the circle on the screen.
Math.py
OUTPUT
Enter the radius of circle: 5
The Perimeter of circle is: 31.41592653589793
3. Using Def() Function:-
In this method, we will define the perimeter of the circle in the def function. As def peri(r) under which we will enter the code of simple way that we learned early. After closing this function we will take radius as input from the user and then type peri(radius) as we have already defined the function of it.
def.py
OUTPUT
Enter the radius of circle: 5.5
The Perimter of circle is: 34.54
Hope this blog post was helpful to you and you have got the answer to your question. Thank you for visiting our blog. If you have any doubts about any coding questions then let us know in the comments section we will answer them as soon as possible. Till then check out our more blog posts.